Understanding Memoization: What is it and when should you use it?
Memoization is a technique that allows you to optimize and speed up programs.
Instead of repeatedly calling a function that performs a slow or expensive calculation, you call it once and store the result. If the function is then called again with the same arguments, it will return the stored result instead of performing the expensive calculation.
By caching results with this technique you can avoid repeated calls to a function that you expect consistent responses from, dramatically increasing the performance of your application as a whole.
Memoization in React
React give you access to the useMemo hook.
By importing and calling useMemo
, you memoize a value so that on subsequent re-renders of the page, the value doesn't have to be recalculated.
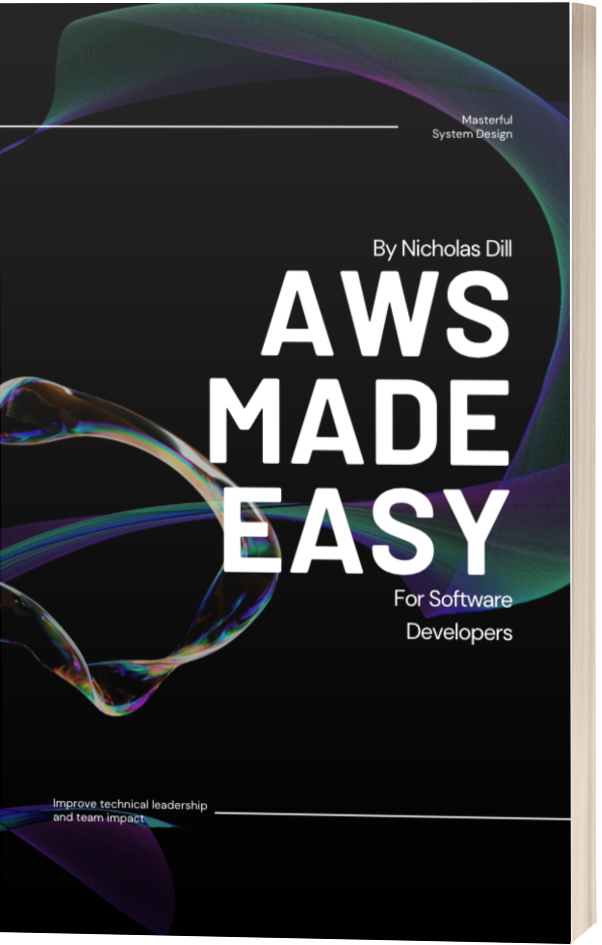
In the below example, we have a simple weather app:
import React, { useMemo } from 'react';
function WeatherApp() {
const weatherForecast = useMemo(() => fetchWeather(), []);
return (
<div>
<p>The weather today is:</p>
<p>{ weatherForecast } </p>
</div>
);
}
It makes a slow API call to fetch the current forecast and it should not frequently change, so we feel okay about memoizing this value.
After the first render, we'll fetch the forecast. But then on the next rerender, we don't make another API call because we stored the response from the first.
Wrapping Up
And that's memoization in a nutshell.
It allows you to save your application from having to repeat calls and duplicate work, greatly increasing the speed and performance of your app as a whole.
If you come across parts of your code that might be duplicating work like this, consider memoization!