Understanding Instance Variables in Ruby on Rails
An instance variable is a variable that belongs to a specific instance of a class.
Every instance of this class can privately store its own unique value. And by default, the instance variable is accessible from anywhere within the class.
This allows us to initialize multiple copies or instances of the same class that can keep their own independent state thanks to instance variables.
Why Use Instance Variables
These types of variables are called instance variables because different instances of the same class can have different values for this variable.
Let's say we wanted to track @points
in a game so we create a class called Scoreboard and initialize 2 instances of this class, one for us and one for our opponent.
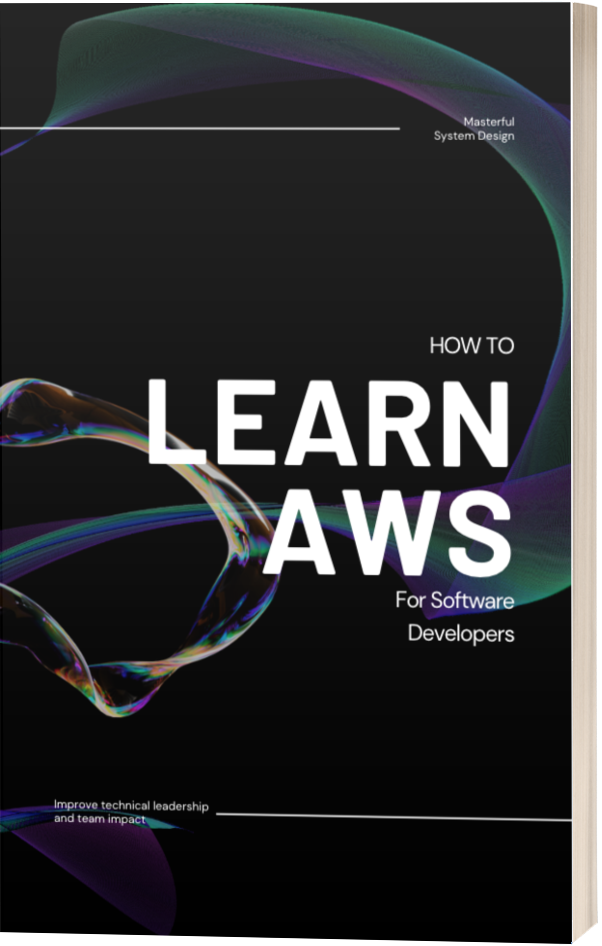
our_team = Scoreboard.new
opponent = Scoreboard.new
Now, thanks to instance variables, we could create some method that is responsible for increasing points and call it on the instance that we intend to increase points for and the other will be unaffected.
>>> puts our_team.points
5
>>> puts opponent.points
0
How to Create Ruby Instance Variables
In Ruby, instance variables are created by prepending an @
to a variable name.
If we created a scoreboard class, we might store points in an instance variable so that this instance is able to track its own score.
class Scoreboard
def initialize
@points = 0
end
end
We can call @points
anywhere else within this class to access the value for this specific instance.
Let's add a method that increases our score.
class Scoreboard
def initialize
@points = 0
end
def score
@points += 5
end
end
If we called the score
method now it would trigger @points += 5
to increase the value of @points
to 5 but only for the specific instance that we called this method on.
So if we called our_team.score
, we would increase the value of @points
only for the our_team
instance and not the opponent
instance.
Accessing Instance Variables Outside of the Class
By default, instance variables are only accessible from inside the class.
Meaning you can't call opponent.@points
or opponent.points
. You can only access and modify @points
directly from inside the Scoreboard
class.
Using Instance Methods
If you want to read the value of an instance you can however create an instance method that reads it from inside the class.
def points
puts @points
end
This would allow us to call opponent.points
because it would call a public method on our class that is accessible from outside the class.
Using Attribute Readers
Another shortcut, Rails has what's called an attribute reader.
That might look like this:
attr_reader :points
It's basically a shorthand version of creating the getter instance method above but it allows you to make instance variables accessible but read-only from outside the class. (You can make them writable too with attribute writers but more on that another time.)
class Scoreboard
attr_reader :points
def initialize
@points = 0
end
def score
@points += 5
end
end
Takeaways
Instance variables privately store a value for a specific instance of a class.
We can initialize multiple copies or instances of the same class that each keep track of their own instance variable values.
We create instance variables by prepending an @
to any variable name.
And we can make them accessible by throwing in an attribute reader on our class like attr_reader :points
.
Hope this helped you understand instance variables!