Routing Multiple Domains in a Ruby on Rails App
A Ruby on Rails app can accept multiple domains or subdomains, and route visitors to different places depending on the domain. This allows me to run multiple projects on the same Rails app, which saves me money and makes it easier to build features that can be shared by multiple projects.
You can wrap a set of resources or endpoints in constraints
where they are only active if the constraints are satisfied, or in this case, if the request is made on a particular domain or subdomain.
Here's an example of how we can route visitors on our shop subdomain:
In our routes.rb
require 'shop_domain'
constraints(ShopDomain) do
resources :shop
get '/subscription', to: 'shop#subscription'
root to: 'shop#index'
end
Then we define our ShopDomain class to filter certain domains or subdomains. I usually place these files in the lib directory.
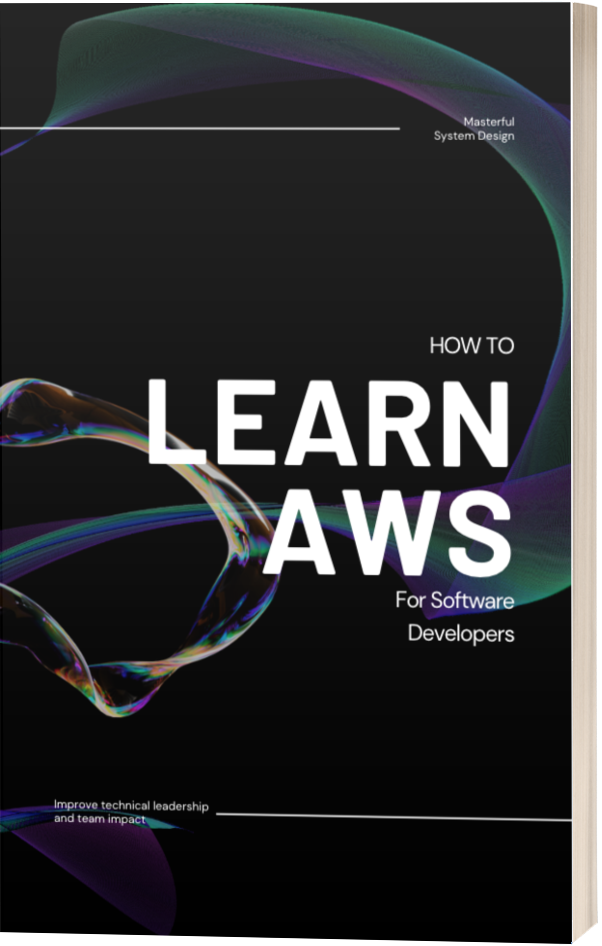
class ShopDomain
def self.matches? request
request.subdomain == 'shop'
end
end
You can easily swap out request.subdomain
for other criteria. I use this trick to map unique domains to their own controllers and views which lets me run a single Rails app that powers multiple websites.
All traffic still hits the same Rails app and all data lives in the same database which makes my life easier.
This approach lets me share code and build a feature for multiple projects simultaneously rather than having to rebuild a feature that I may want in every separate app I develop.
Plus this approach is way more cost-effective than spinning up separate servers for each website.