Stateless Function Components in React
A stateless function component is a typical React component that is defined as a function that does not manage any state.
There are no constructors needed, no classes to initialize, and no lifecycle hooks to worry about.
These functions simply take props as an input and return JSX as an output.
They can be as simple as this:
const Title = ({ title }) => {
return <h1>{title}</h1>;
};
The above example is an arrow function component which let's us write the clearest and most concise react component possible.
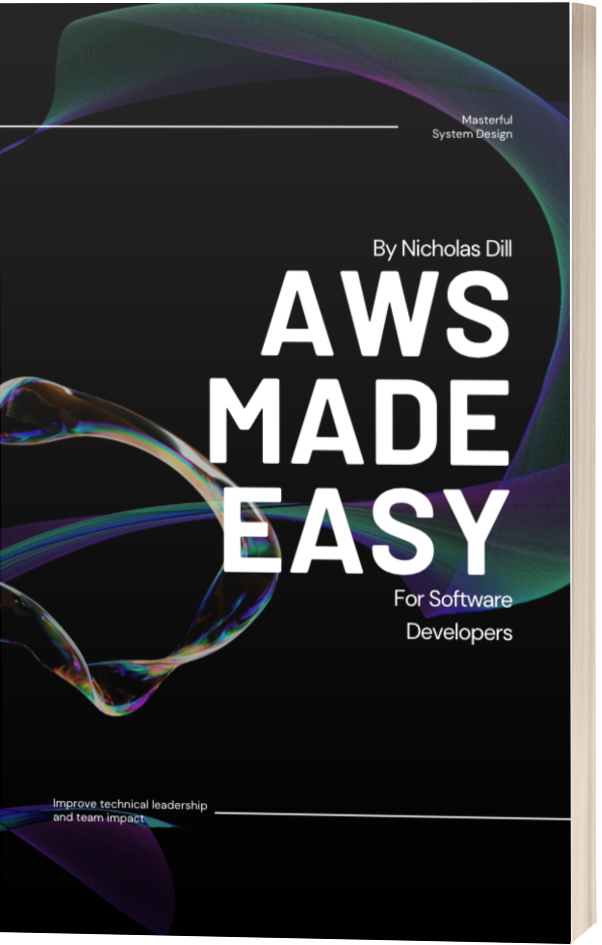
Stateless function components are really nice to work with and I'm a big fan of them because these components have consistent output based on their inputs since they have no state or side-effects.
If you give a stateless function component a set of props it will always render the same JSX.
This differs from a stateful component where state can affect the JSX output, even if the same inputs are given.
Why Use Function Components?
Breaking up your React code into stateless function components can help in many ways.
It's easier to test a stateless function component since there are fewer unexpected outputs. The JSX should always be consistent when you are given an input.
You don't have to define so many things. There is significantly less boilerplate when creating function components.
Also, these components offer better readability and an easier way to create new components. Other developers can understand functions without needing to understand the inherited class methods and logic of traditional class components. In other words, no need to extend React.Component
or have any knowledge around what calls the render()
method.
I like this concept, but I still need states!
You can combine this approach with traditional stateful components to get the best of both worlds.
React Hooks make it possible to add state to Function Components. There is a specific hook called the useState hook that manages state in your component with a single line of code. Goodbye class component boilerplate!
And since you no longer have access to traditional React lifecycle methods like onComponentDidMount
, you can achieve almost all of the same logic by taking advantage of React's useEffect hook.
Hooks are a big improvement that have made it easier to learn and work with React in general.
I hope this helped explain how you can take advantage of functional components in React!